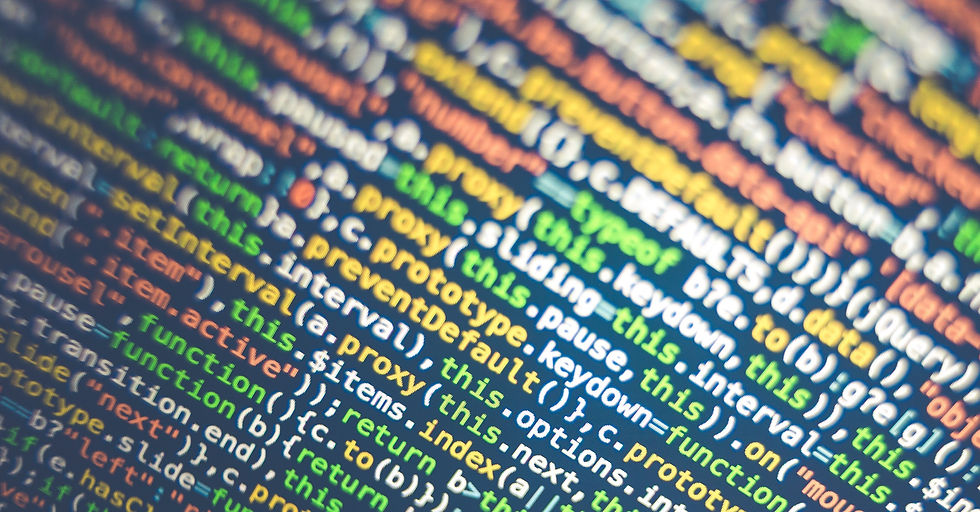
One of the fundamental concepts in Python is data types, which are the building blocks for all programs. In this article, we will discuss the various basic data types used within Python and provide examples of how they are used in programming.
Integers are whole numbers, such as 1, 2, 3, 4, and so on. In Python, integers have no decimal point and can be positive or negative. They are used to represent quantities that can be counted, such as the number of apples in a basket or the number of days in a week. Here's an example of how to define an integer variable in Python:
x = 5
Floating-point numbers are decimal numbers, such as 3.14, 2.5, and 6.75. In Python, floating-point numbers are represented using the float data type. They are used to represent quantities that can be measured, such as the temperature, the weight of an object, or the distance traveled. Here's an example of how to define a floating-point variable in Python:
y = 3.14
Booleans are binary values that can be either true or false. In Python, Booleans are represented using the bool data type. They are used in programming to represent conditions, such as whether a statement is true or false. Here's an example of how to define a Boolean variable in Python:
z = True
Strings are sequences of characters, such as "hello", "world", and "Python". In Python, strings are represented using the str data type. They are used to represent text or messages in programming. Here's an example of how to define a string variable in Python:
message = "Hello, world!"
Lists are ordered collections of elements, such as [1, 2, 3], ["apple", "banana", "orange"], and [True, False, True]. In Python, lists are represented using the list data type. They are used to store a group of related values or data items. Here's an example of how to define a list variable in Python:
my_list = [1, 2, 3, 4, 5]
Tuples are ordered collections of elements, similar to lists. However, unlike lists, tuples are immutable, meaning that their contents cannot be modified once they are created. In Python, tuples are represented using the tuple data type. They are used to store related values or data items that should not be modified. Here's an example of how to define a tuple variable in Python:
my_tuple = (1, 2, 3, 4, 5)
Sets are unordered collections of unique elements, such as {1, 2, 3}, {"apple", "banana", "orange"}, and {True, False}. In Python, sets are represented using the set data type. They are used to store a group of unique values or data items. Here's an example of how to define a set variable in Python:
my_set = {1, 2, 3, 4, 5}
Dictionaries are unordered collections of key-value pairs, such as {"name": "John", "age": 30}, {"fruit": "apple", "color": "red"}, and {"status": "married", "children": 2}.
my_dict = {"name": "John", "age": 30, "city": "New York"}
Comments