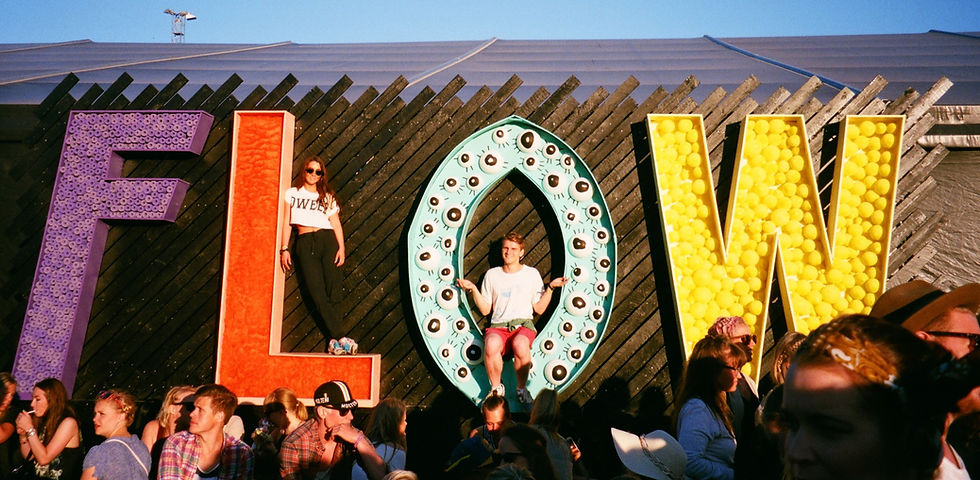
Control flow statements are an essential aspect of programming, as they allow developers to create more complex and dynamic programs. In this article, we will discuss the various control flow statements that are available in Python and provide examples to illustrate how they work.
if statement
The if statement is used to execute a block of code if a condition is true. The syntax for the if statement is as follows:
if condition: # execute code if condition is true
For example, suppose we want to check if a given number is positive or negative. We can use the following code:
num = 5
if num > 0:
print("Number is positive")
In this example, if the value of the variable num is greater than 0, the message "Number is positive" will be printed.
if-else statement
The if-else statement is used to execute a block of code if a condition is true, and another block of code if the condition is false. The syntax for the if-else statement is as follows:
if condition: # execute code if condition is true
else: # execute code if condition is false
For example, suppose we want to check if a given number is even or odd. We can use the following code:
num = 4
if num % 2 == 0:
print("Number is even")
else:
print("Number is odd")
In this example, if the value of the variable num is divisible by 2 (i.e., it is even), the message "Number is even" will be printed. Otherwise, the message "Number is odd" will be printed.
elif statement
The elif statement is used to check multiple conditions and execute a block of code based on the condition that is true. The syntax for the elif statement is as follows:
if condition1: # execute code if condition1 is true
elif condition2: # execute code if condition2 is true
else: # execute code if all conditions are false
For example, suppose we want to check if a given number is positive, negative, or zero. We can use the following code:
num = -3
if num > 0:
print("Number is positive")
elif num < 0:
print("Number is negative")
else: print("Number is zero")
In this example, if the value of the variable num is greater than 0, the message "Number is positive" will be printed. If the value of num is less than 0, the message "Number is negative" will be printed. Otherwise, the message "Number is zero" will be printed.
for loop
The for loop is used to iterate over a sequence (such as a list or a string) and execute a block of code for each element in the sequence. The syntax for the for loop is as follows:
for variable in sequence: # execute code for each element in sequence
For example, suppose we want to print each character in a given string. We can use the following code:
string = "Hello, world!"
for char in string:
print(char)
In this example, the for loop iterates over each character in the string and prints it to the console.
while loop
The while loop is used to execute a block of code as long as a condition is true. The syntax for the while loop is as follows:
a, b = 0
count = 0
while count < 10:
print(a)
c = a + b
a, b = b, c
count += 1
In this example, we first initialize the variables a and b to 0 and 1, respectively, and set count to 0. We then use a while loop to print out the first 10 numbers in the Fibonacci sequence.
The while loop continues to execute as long as the count variable is less than 10. Inside the loop, we first print out the current value of a. We then calculate the next value in the sequence by adding a and b together and storing the result in c. We then update a to be equal to b, and b to be equal to c.
Finally, we increment the value of count by 1, which allows the loop to eventually terminate after 10 iterations.
When we run this code, it should output the following:
0 1 1 2 3 5 8 13 21 34
These are the first 10 numbers in the Fibonacci sequence, which is a series of numbers in which each number is the sum of the two preceding numbers.
Comments