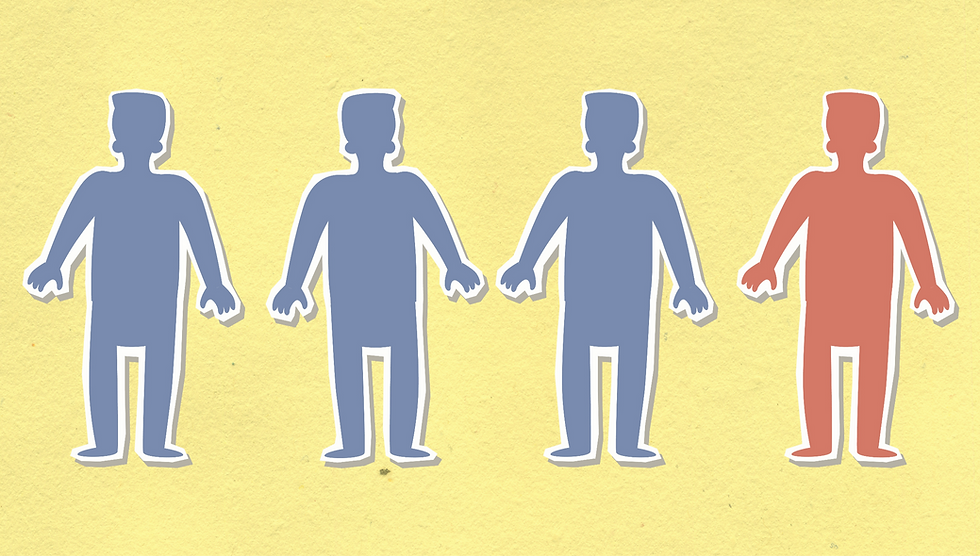
What are Exceptions in Python?
An exception is an event that interrupts the normal flow of a program's execution. When an exception occurs, the program's current execution path is halted, and control is transferred to a special error-handling routine called an exception handler. The exception handler can then perform whatever actions are necessary to address the exception and resume normal program execution.
In Python, exceptions are implemented as objects that are derived from the built-in BaseException class. There are many different types of exceptions in Python, each of which is designed to handle a specific type of error or exceptional situation.
How to Handle Exceptions in Python
When an exception occurs in Python, the interpreter looks for an exception handler that can handle the exception. If no appropriate handler is found, the program will terminate and an error message will be displayed.
To handle exceptions in Python, you can use a try statement. A try statement consists of a try block, which contains the code that might raise an exception, and one or more except blocks, which contain the code that will handle the exception if it occurs. Here's a simple example:
try:
x = 1 / 0
except ZeroDivisionError:
print("Error: division by zero")
In this example, the try block attempts to divide the number 1 by 0, which would raise a ZeroDivisionError exception. The except block then handles the exception by printing an error message.
In addition to try and except, Python also provides several other keywords and constructs that can be used to handle exceptions, including finally, else, and raise. These are used to control the flow of exception handling and provide additional functionality for handling complex error scenarios.
Common Examples of Python Exceptions
Let's take a look at some common examples of exceptions in Python, and how they can be handled using exception handling techniques.
IndexError
The IndexError exception is raised when you try to access an index of a list, tuple, or other sequence that is out of range. Here's an example:
my_list = [1, 2, 3]
try:
print(my_list[3])
except IndexError:
print("Error: index out of range")
In this example, the try block attempts to access the fourth element of my_list, which doesn't exist, causing an IndexError exception to be raised. The except block then handles the exception by printing an error message.
KeyError
The KeyError exception is raised when you try to access a key in a dictionary that doesn't exist. Here's an example:
my_dict = {"key1": "value1", "key2": "value2"}
try:
print(my_dict["key3"])
exceptKeyError:
print("Error: key not found")
In this example, the try block attempts to access the value associated with the key "key3", which doesn't exist in my_dict, causing a KeyError exception to be raised. The except block then handles the exception by printing an error message.
ValueError
The ValueError exception is raised when you pass an invalid value to a function or method. Here's an example:
pythonCopy code
try: int("abc") except ValueError: print("Error: invalid literal for int() with base 10")
In this example, the try block attempts to convert the string "abc" to an integer using the int() function, which is not a valid integer value, causing a ValueError exception to be raised. The except block then handles the exception by printing an error message.
FileNotFoundError
The FileNotFoundError exception is raised when you try to access a file that doesn't exist or can't be opened. Here's an example:
try:
with open("nonexistent_file.txt", "r") as f:
print(f.read())
exceptFileNotFoundError:
print("Error: file not found")
In this example, the try block attempts to open a file called "nonexistent_file.txt" for reading using the open() function, which doesn't exist in the current directory, causing a FileNotFoundError exception to be raised. The except block then handles the exception by printing an error message.
TypeError
The TypeError exception is raised when you pass an object of the wrong type to a function or method. Here's an example:
try:
len(1)
except
TypeError: print("Error: object of type 'int' has no len()")
In this example, the try block attempts to get the length of the integer value 1 using the len() function, which only works with sequences like strings and lists, causing a TypeError exception to be raised. The except block then handles the exception by printing an error message.
KeyboardInterrupt
The KeyboardInterrupt exception is raised when the user interrupts the program by pressing Ctrl+C. Here's an example:
try:
while True:
pass
except KeyboardInterrupt:
print("Program interrupted by user")
In this example, the try block creates an infinite loop that can only be interrupted by the user pressing Ctrl+C, causing a KeyboardInterrupt exception to be raised. The except block then handles the exception by printing an error message.
Conclusion
In conclusion, exceptions are an essential tool for handling errors and unexpected events in Python. By using try and except statements, you can handle a wide variety of exceptions and prevent your program from crashing or behaving unpredictably. By understanding the different types of exceptions and how to handle them, you can write more robust and reliable code.
Comments